Related Topics
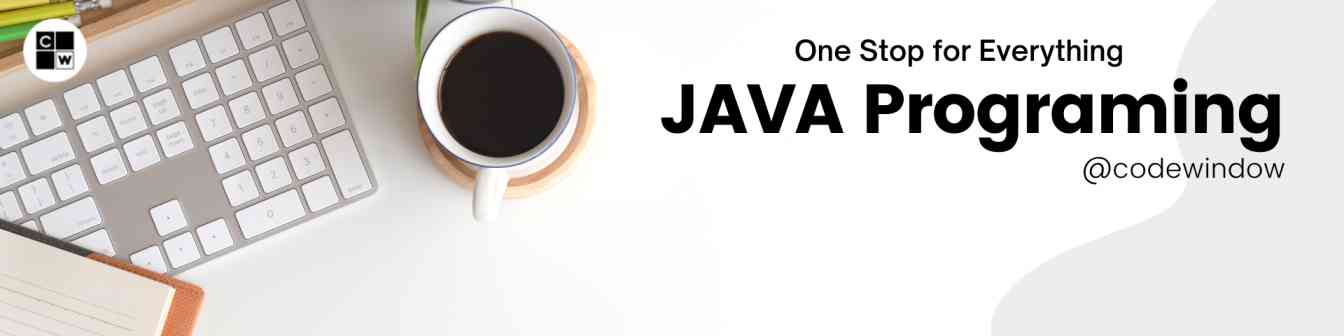
JAVA Programming
Assignment operators are used to assign values to variables. For example:
Comparison operators are used to compare values and return a boolean result (true or false). For example:
Logical operators are used to perform logical operations on boolean values. For example:
Bitwise operators are used to perform operations on the binary representations of integer values. For example:
Assignment Operators: These operators are used to assign a value to a variable. The assignment operator is
=
and it can be combined with other arithmetic operators to create shorthand assignments such as+=
,-=
and so on.
Examples:
Comparison Operators: These operators are used to compare two values and return a boolean result (
true
orfalse
). The comparison operators are<
,>
,<=
,>=
,==
and!=
.
Examples:
Logical Operators: These operators are used to combine boolean expressions and return a boolean result. The logical operators are
&&
(logical AND),||
(logical OR) and!
(logical NOT).
Examples:
Bitwise Operators: These operators are used to perform bitwise operations on integer values. The bitwise operators are
&
(bitwise AND),|
(bitwise OR),^
(bitwise XOR),~
(bitwise NOT),<<
(left shift),>>
(right shift) and>>>
(unsigned right shift).
Examples:
In this example, we have two variables x and y, and we’re performing different arithmetic operations on them using the arithmetic operators.
The
+
operator is used to add x and y, which results in the sum of 13.The
-
operator is used to subtract y from x, which results in the difference of 7.The
*
operator is used to multiply x and y, which results in the product of 30.The
/
operator is used to divide x by y, which results in the quotient of 3.The
%
operator is used to find the remainder of x divided by y, which results in the remainder of 1.




Popular Category
Topics for You
Go through our study material. Your Job is awaiting.